Big Button - Part 9 - Rust Development Workflow
Wed, Feb 12, 2025
|5 min read
|
Table of Contents
Introduction
💻 HACKER In the previous steps we simply edited files with pre-defefined code, before running some commands in the terminal. This bare workflow may work, however there are some tools and methods that we can use to help us develop in Rust.
Here we attempt to outline these tools and methods. The goal here is to show how to add, test, and iterate Rust code for this project.
TIP
Remember, a software development workflow can be subjective and ever-evolving, where each developer may have his/her preferences, settings, and so on. Being relatively new to Rust embedded development, my advice is to first follow what is outlined here, then try to customize and set your workflow however fits you.
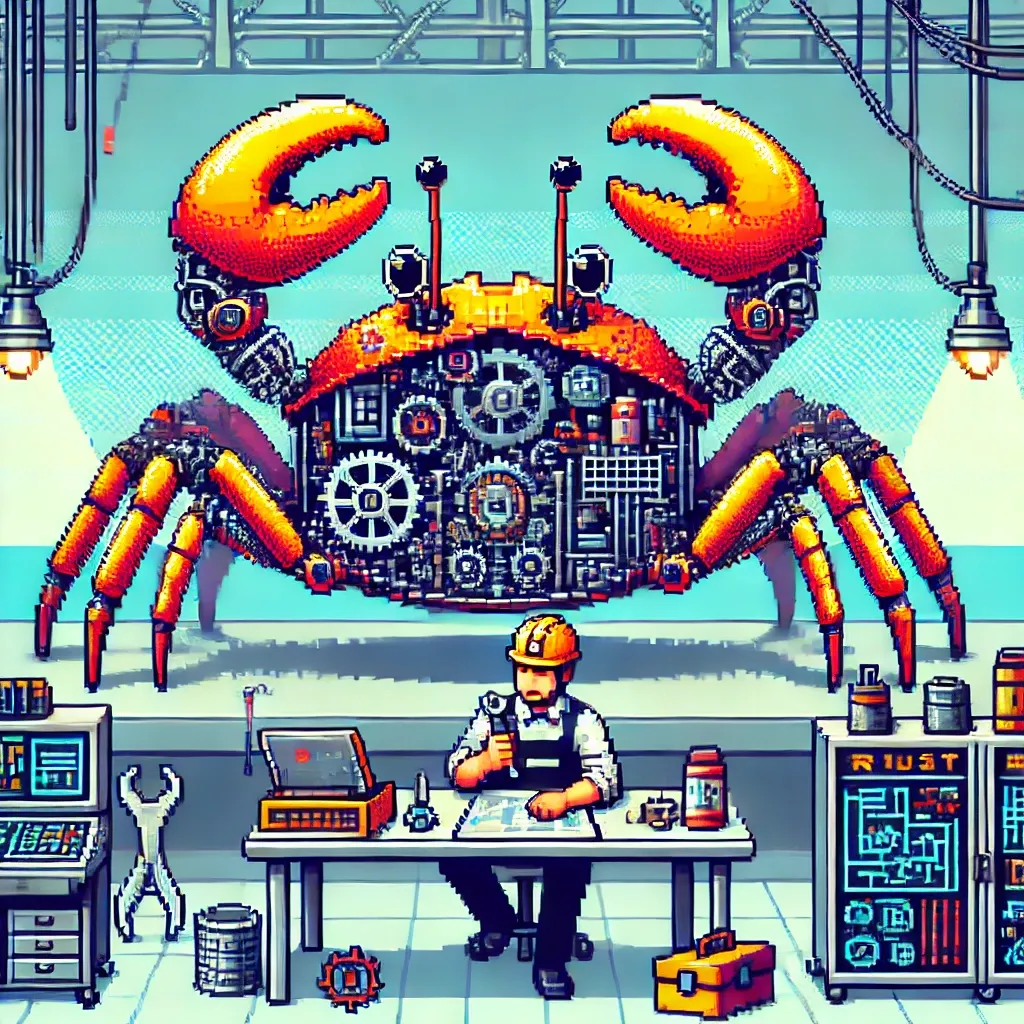
Tools
Add the following tools to your local toolbelt on your computer. For each tool, we will briefly mention the tool, outline some notes, and define its importance and usage.
rust-analyzer
This tool is the official Language Server Protocol (LSP)
server for Rust that provides code completion and
code navigation capabilities. Aside from the fundamental Rust tools like cargo
, rust-analyzer
is essential for effective development.
rust-analyzer
typicall runs in the background and parallel with your IDE, constantly watching
your keystrokes and code changes. When appropriate, it provides auto-completion
hints and options, while also enabling you and your IDE to effectively navigate your
code.
Official documentation for rust-analyzer
can be found here.
To install rust-analyzer
, follow these links:
After installation, rust-analyzer
is enabled and started by default when working on Rust code.
bacon
🥓
This tool is a background code checker/linter for Rust that provides real-time error detection and
warnings with minimal user interaction. bacon
runs continuously
in a separate terminal, providing immediate feedback as you code.
It’s highly customizable and integrates
seamlessly with your development workflow. Official documentation for bacon
can be found here.
To install bacon
, execute the following:
cargo install --locked bacon
After installation, you can run the following command within the project
root directory (Stop it with pressing q
):
bacon --all-features --job clippy
TIP
probe-rs
offers a Visual Studio Code extension
that can use the probe-rs
CLI tool with VS Code to offer more advanced debugging and monitoring capabilities.
In this tutorial we will not be focused on using this extension, however I encourage
you to familiarise yourself with it and see if that is something you are interested in.
Logging Messages
While logging statments may not be the most powerful or most sexy debugging tool, they are effective, simple to understand and use, and tried and true!
The concept is simple. Any time you need to see some information at a specific point in your code, you add a logging statment which will show that infromation in the terminal output during the program’s run.
Specifically speaking, we will use defmt
with the following syntax to output logging.
// Import the following on top of the fileuse defmt::*; // Import all items from defmtuse defmt_rtt as _; // Import defmt_rtt - Real-Time Transfer (RTT) as transport for defmt loguse panic_probe as _; // Ensure that panic messages are send through the debug probe
let my_variable = 3;
// Use the various logging functionsdebug!("A debug level logging message");info!("This is a simple info level log message");error!("This message has a variable: {}", my_variable);
For our configured project, the output for this specific example Rust code would look like this:
[DEBUG ] example.rs:9 : A debug level logging message[INFO ] example.rs:10 : This is a simple info level log message[ERROR ] example.rs:11 : This message has a variable: 3
The Workflow ♻️
Setup
-
Open the project’s root directory in the IDE.
-
Open up the first terminal for code warnings/error monitoring.
-
Run the following command in this first terminal:
Terminal window bacon --all-features --job clippy -
Open up a second terminal for flashing and running our code.
Add Changes Continuously
-
Using your IDE, make some changes to the code or project configurations.
-
Monitor if those changes raised any warnings or errors in the
bacon
terminal. -
In second terminal used for flashing and running code, run the following:
Terminal window cargo run --release -
Monitor logs, the controller, and all the things …
-
Stop this run with pressing
Ctrl-c
-
Repeat steps until you are done with your task
Commit Changes (Saving Your Work)
-
Use
git
to add/stage your changes..Terminal window git add . -
Commit changes with a message. Feel free to use conventional git commit messages.
Terminal window git commit -m "did some fun work" -
Push the changes to GitHub.
Terminal window git push origin main
That’ll be our workflow. Now let’s add more CODE!