Big Button - Part 5 - Programming Hardware Setup and Test
Mon, Jan 6, 2025
|7 min read
|
Table of Contents
Introduction
Here we are finally doing some computer software stuff!💻 HACKER We are going to install all software needed to start interacting with our Raspberry Pi Pico W microcontroller. We will setup everything for a simple connection smoke test, verifying things are ready to go for further programming and subsequent steps.
That is, we are explaining and setting up:
- Rust and Rust programming tools
- Debugging and Target Interaction Toolkit
Rust
This is the part where we get all tools needed to start developing in the Rust programming language.
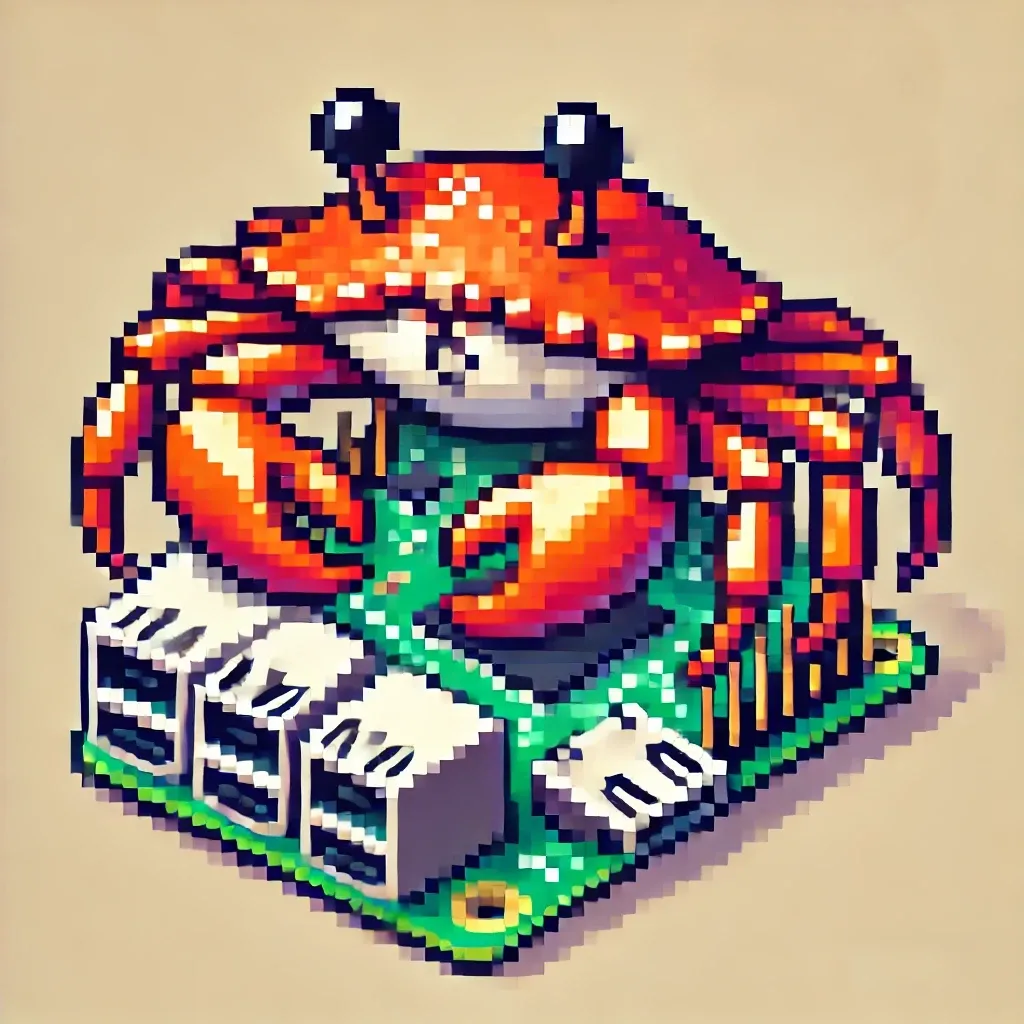
About Rust
Rust is a systems programming language that emphasizes safety, concurrency, and memory efficiency. It achieves these goals through a unique ownership system, a rich type system, and fearless concurrency primitives. Basically, it is very good for low level programming.
In terms of programming embedded devices, Rust is great because of the following.
- Rust provides low-level control: Efficiently manage memory and hardware without an OS or runtime.
- Memory safety: Eliminate entire classes of bugs like null pointer dereferences and data races.
- Zero-cost abstraction: Write clean high-level interfaces that compile to optimized machine code.
- Fast growing ecosystem: Take advantage of a rapidly expanding set of libraries, tools, and frameworks for embedded Rust.
- Rust is fast!
More information on Rust and embedded programming found here.
Installing Rust
Once Rust is installed, we will have a few Rust command line interface (CLI) tools available. Before installing anything, let’s review what each installed Rust tool is used for.
-
rustup
: Manages Rust versions and associated tools. It is used to install, update, and switch between Rust toolchains. Here is way more information about this tool within therustup
book. Note that a Rust toolchain is a single installation of the Rust compiler and associated tools. You can have multiple toolchains depending on the environment or project. -
cargo
: Handles dependencies, runs tests, generates documentation, and more. This is the de facto way to manage Rust projects. This is the most used Rust CLI tool. There is lots more information on this tool found in thecargo
book -
rustc
: The Rust compiler that transforms your written code into an executable/binary. This command is typically invoked viacargo
rather than directly. You will often not use this exclusively by itself.
To check if rust is already installed. Open up your computer terminal and enter the following command. If you do not get a version number, you do not have Rust installed and will have to install it.
rustc --version
The official Rust installation instructions are very good. The installation instructions can be found there.
If you do have Rust installed, feel free to go ahead and update all your local Rust toolchains
rustup update
NOTE
For MacOS and Linux, this should be one single and simple command. However, if you are on
a Windows computer, please visit rustup.rs and download and
run the rustup-init.exe
.
Debugging and Target Interaction Toolkit
About
When writing code for embedded devices like the Raspberry Pi Pico, you will need to load code/program/instructions onto the target processor, control its execution, examine and modify memory contents, and receive debugging information.
A Debugging and Target Interaction Toolkit are programs that provide a set of standardized interfaces and protocols to facilitate this low-level interaction between the development host computer and the target embedded system.
This includes:
- Flashing microcontroller firmware
- Reading and writing memory, running, halting, setting and reading breakpoints
- Getting logs from the microcontroller
probe-rs
probe-rs
is a embedded debugging and target interaction toolkit. It enables its user to
program and debug microcontrollers via a debug probe device.
Essentially, the interiaction between your program/code and the microcontroller looks like this:
- Your super cool program/code
probe-rs
software- USB protocol for hardware (ie. CMSIS-DAP, ST-Link, J-Link, FTDI)
- Debug probe hardware
- Raspberry Pi Pico W Microcontroller
See more information about the probe-rs
tool here.
NOTE
Note that probe-rs
is not the only tool for debugging and flashing. Other tools
include OpenOCD and PyOCD.
probo-rs
Install and Setup
The following are install methods and scripts that are found in the official probe-rs
documentation.
If you are interested in more details or other ways to install this tool
consult the official website.
MacOS
Install using Homebrew package manager:
brew tap probe-rs/probe-rsbrew install probe-rs
… or using the official installer script:
curl --proto '=https' --tlsv1.2 -LsSf https://github.com/probe-rs/probe-rs/releases/latest/download/probe-rs-tools-installer.sh | sh
Windows
Install using the installer script for Windows pre-installed PowerShell terminal
with the irm
(Invoke-RestMethod
)
and iex
(Invoke-Expression
)
irm https://github.com/probe-rs/probe-rs/releases/latest/download/probe-rs-tools-installer.ps1 | iex
Linux
Install using the official installer script:
curl --proto '=https' --tlsv1.2 -LsSf https://github.com/probe-rs/probe-rs/releases/latest/download/probe-rs-tools-installer.sh | sh
For Linux you will have to set up Userspace Device Management (udev) rules for our debug probe seperately. udev rules are instructions that tell Linux what to do when specific devices (like USB drives or debug probes) are plugged into your computer. These rules files control things like who can access the device, what name it gets, and what automatic actions should happen when the device is connected
Execute the following commands in your terminal:
# Download the udev rule into /tmp/curl -o /tmp/69-probe-rs.rules https://probe.rs/files/69-probe-rs.rules
# Move the udev rule file to its system directorysudo cp /tmp/69-probe-rs.rules /etc/udev/rules.d/
# Reload and apply all udev rules to connected devicessudo udevadm control --reloadsudo udevadm trigger
Verify Debug Probe
Let’s verify that our setup worked by connecting our debug probe device to the computers USB port. For now, don’t worry about the Raspberry Pi Pico W, we are just checking if we can talk to the debug probe.
After connecting the debug probe, run the following command.
probe-rs list
You should see something like the following.
The following debug probes were found:[0]: Debug Probe (CMSIS-DAP) -- 2e8a:000c:E6633861A3125F2C (CMSIS-DAP)
If you see such and output, great! You are done with this section. If you do not see that, try some of the follwoing steps.
- Verify all hardware is connected and powered (usb port, debug probe)
- Verify the USB port is properly working
- All of the listed commands executed successfully
References
- Rust Programming Language
- Rust Embedded Device Programming Info
- Installing Rust
- probe-rs CLI tool
- Overview to udev Rules for Linux
- Getting Started with Embedded Rust, Embassy, and the Raspberry Pi Pico
Cool. Next we will setup and test our programming environment …